最简单的jQuery轮播图
2023-07-08 03:49
代码描述:自写jQuery轮播图
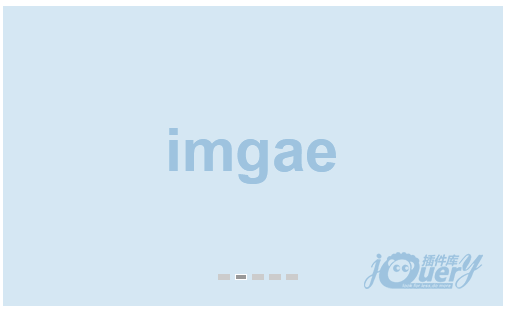
HTML
<div class="middle_right">
<div id="lunbobox">
<div id="toleft"><</div>
<div class="lunbo">
<a href="#"><img src="http://www.jq22.com/img/cs/500x300a.png" /></a>
<a href="#"><img src="http://www.jq22.com/img/cs/500x300b.png" /></a>
<a href="#"><img src="http://www.jq22.com/img/cs/500x300c.png" /></a>
<a href="#"><img src="http://www.jq22.com/img/cs/500x300d.png" /></a>
<a href="#"><img src="http://www.jq22.com/img/cs/500x300.png" /></a>
</div>
<div id="toright">></div>
<ul>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
<span></span>
</div>
</div>
CSS
#lunbobox {
width: 500px;
height: 300px;
position: relative;
}
.lunbo {
width: 500px;
height: 300px;
}
.lunbo img {
width: 500px;
height: 300px;
position: absolute;
top: 0px;
left: 0px;
}
#lunbobox ul {
width: 285px;
position: absolute;
bottom: 10px;
right: 0px;
z-index: 5;
}
#lunbobox ul li {
cursor: pointer;
width: 10px;
height: 4px;
border: 1px solid #cccccc;
float: left;
list-style: none;
background: #cccccc;
text-align: center;
margin: 0px 5px 0px 0px;
}
#toleft {
display: none;
width: 30px;
height: 100px;
font-size: 40px;
line-height: 100px;
text-align: center;
color: #f4f4f4;
/*background:#cccccc;
*/
/*background:url("../images/toleft.jpg")no-repeat center;
*/
position: absolute;
top: 90px;
left: 12px;
cursor: pointer;
z-index: 99;
opacity: 0.4;
}
#toright {
display: none;
width: 30px;
height: 100px;
font-size: 40px;
line-height: 100px;
text-align: center;
color: #f4f4f4;
/*background:#cccccc;
*/
position: absolute;
top: 90px;
right: 0px;
cursor: pointer;
z-index: 99;
opacity: 0.4;
}
JS
///轮播
$(function () {
//$("#toright").hide();
//$("#toleft").hide();
$("#toright").hover(
function () {
$("#toleft").hide();
},
function () {
$("#toleft").show();
}
);
$("#toleft").hover(
function () {
$("#toright").hide();
},
function () {
$("#toright").show();
}
);
});
var t;
var index = 0;
/////自动播放
t = setInterval(play, 3000);
function play() {
index++;
if (index > 4) {
index = 0;
}
// console.log(index)
$("#lunbobox ul li")
.eq(index)
.css({
background: "#999",
border: "1px solid #ffffff"
})
.siblings()
.css({
background: "#cccccc",
border: ""
});
$(".lunbo a ").eq(index).fadeIn(1000).siblings().fadeOut(1000);
}
///点击鼠标 图片切换
$("#lunbobox ul li").click(function () {
//添加 移除样式
//$(this).addClass("lito").siblings().removeClass("lito"); //给当前鼠标移动到的li增加样式 且其余兄弟元素移除样式 可以在样式中 用hover 来对li 实现
$(this)
.css({
background: "#999",
border: "1px solid #ffffff"
})
.siblings()
.css({
background: "#cccccc"
});
var index = $(this).index(); //获取索引 图片索引与按钮的索引是一一对应的
// console.log(index);
$(".lunbo a ").eq(index).fadeIn(1000).siblings().fadeOut(1000); // siblings 找到 兄弟节点(不包括自己)
});
/////////////上一张、下一张切换
$("#toleft").click(function () {
index--;
if (index <= 0) {
//判断index<0的情况为:开始点击#toright index=0时 再点击 #toleft 为负数了 会出错
index = 4;
}
console.log(index);
$("#lunbobox ul li")
.eq(index)
.css({
background: "#999",
border: "1px solid #ffffff"
})
.siblings()
.css({
background: "#cccccc"
});
$(".lunbo a ").eq(index).fadeIn(1000).siblings().fadeOut(1000); // siblings 找到 兄弟节点(不包括自己)必须要写
}); // $("#imgbox a ")获取到的是一个数组集合 。所以可以用index来控制切换
$("#toright").click(function () {
index++;
if (index > 4) {
index = 0;
}
console.log(index);
$(this).css({
opacity: "0.5"
});
$("#lunbobox ul li")
.eq(index)
.css({
background: "#999",
border: "1px solid #ffffff"
})
.siblings()
.css({
background: "#cccccc"
});
$(".lunbo a ").eq(index).fadeIn(1000).siblings().fadeOut(1000); // siblings 找到 兄弟节点(不包括自己)
});
$("#toleft,#toright").hover(
function () {
$(this).css({
color: "black"
});
},
function () {
$(this).css({
opacity: "0.3",
color: ""
});
}
);
///
///////鼠标移进 移出
$("#lunbobox ul li,.lunbo a img,#toright,#toleft ").hover(
////////鼠标移进
function () {
$("#toright,#toleft").show();
clearInterval(t);
},
///////鼠标移开
function () {
//$('#toright,#toleft').hide()
//alert('aaa')
t = setInterval(play, 3000);
function play() {
index++;
if (index > 4) {
index = 0;
}
$("#lunbobox ul li")
.eq(index)
.css({
background: "#999",
border: "1px solid #ffffff"
})
.siblings()
.css({
background: "#cccccc"
});
$(".lunbo a ").eq(index).fadeIn(1000).siblings().fadeOut(1000);
}
}
);