前端经典案例之轮播图
2023-07-18 06:24
大部分网站或APP的首页差不多都运用到了轮播图,下边就探讨一下关于轮播图的集中解决方法,相关插件可自行去网站下载。
效果图:
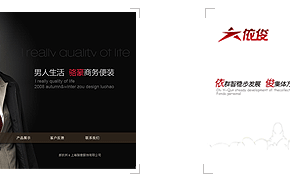
方法一:
1.css样式代码:
<style>
div {
width: 344px;
height: 199px;
overflow: hidden;
}
ul {
width: 1042px;
}
li {
display: inline-block;
}
</style>
2.html代码:
<div class="wrapper">
<ul>
<li><img src="07.gif" /></li>
<li><img src="08.gif" /></li>
<li><img src="09.gif" /></li>
</ul>
</div>
3.js代码:
<script src="http://apps.bdimg.com/libs/jquery/1.11.1/jquery.js"></script>
<script>
var imgWidth = $(".wrapper").width(); //ul移动一次的距离
var imgLength = $("ul li").length; //判断索引为最后一个时,重新置为0
var index = 0; //索引
function changeImg(direction) {
if (direction == "left") {
index++;
} else {
index--;
}
if (index == imgLength) {
index = 0;
} else if (index == -1) {
index = imgLength - 1;
}
$("ul")
.stop()
.animate(
{
marginLeft: -index * imgWidth + "px"
},
600
);
}
function autoMove() {
setInterval(function () {
changeImg("left");
}, 2000);
}
autoMove();
</script>
方法二:
效果图:
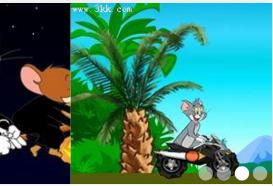
注释:该方法实现的轮播图是可循环的。
1.css代码:
.wrapper {
width: 100%;
height: 200px;
overflow: hidden;
position: absolute;
}
.wrapper ul {
width: 400%;
height: 200px;
font-size: 0;
position: relative;
}
.wrapper ul li {
display: inline-block;
}
.wrapper img {
width: 100%;
height: 200px;
}
.wrapper .dots {
position: absolute;
right: 5px;
top: 180px;
}
.wrapper .dots span {
width: 16px;
height: 16px;
display: inline-block;
background: #FFF;
opacity: .5;
border-radius: 50%;
}
2.HTML代码:
<div class="wrapper">
<ul>
<li><img src="img/4-1.jpg" /></li>
<li><img src="img/4-2.jpg" /></li>
<li><img src="img/4-3.jpg" /></li>
<li><img src="img/4-4.jpg" /></li>
</ul>
<div class="dots">
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</div>
3.js代码:
<script src="js/zepto.js"></script>
<script>
var index = 1;
var liLnegth = $("li").length;
$(".dots span").eq(0).css("opacity", "1");
setInterval(function () {
$("ul").css("left", "0%");
$("ul").animate(
{
left: -100 + "%"
},
1000
);
$(".dots span")
.eq(index - 1)
.css("opacity", ".5");
$(".dots span").eq(index).css("opacity", "1");
$("ul li").eq(0).remove().clone(true).appendTo($("ul"));
index++;
if (index == liLnegth) {
index = 0;
}
}, 2000);
</script>
方法三:
借助touchSlide插件 注释:该方法在移动端可以手动左右滑动,但是不可循环
1.css代码:
.focus {
width: 100%;
height: 140px;
margin: 0 auto;
position: relative;
overflow: hidden;
}
.focus .hd {
width: 100%;
height: 11px;
position: absolute;
z-index: 1;
bottom: 5px;
text-align: center;
}
.focus .hd ul {
display: inline-block;
height: 5px;
padding: 3px 5px;
background-color: rgba(255, 255, 255, 0.7);
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
border-radius: 5px;
font-size: 0;
vertical-align: top;
}
.focus .hd ul li {
display: inline-block;
width: 5px;
height: 5px;
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
border-radius: 5px;
background: #8C8C8C;
margin: 0 5px;
vertical-align: top;
overflow: hidden;
}
.focus .hd ul .on {
background: #FE6C9C;
}
.focus .bd {
position: relative;
z-index: 0;
}
.focus .bd li img {
width: 100%;
height: 140px;
}
/* 修改 */
.focus .hd {
text-align: right;
bottom: 10px;
}
.focus .hd ul {
background-color: transparent;
}
.focus .hd ul li {
width: 10px;
height: 10px;
-webkit-border-radius: 10px;
opacity: 0.6;
}
.focus .hd ul .on {
background: #fff;
opacity: 1;
}
注释:css代码部分有所修改
2.html代码:
<div id="banner" class="focus">
<div class="hd">
<ul></ul>
</div>
<div class="bd">
<ul>
<li>
<a href="#"><img _src="img/商城banner.jpg" /></a>
</li>
<li>
<a href="#"><img _src="img/商城banner.jpg" /></a>
</li>
<li>
<a href="#"><img _src="img/商城banner.jpg" /></a>
</li>
</ul>
</div>
</div>
3.js代码:
<script type="text/javascript" src="js/zepto.js"></script>
<script type="text/javascript" src="js/TouchSlide.1.1.js"></script>
<script type="text/javascript">
TouchSlide({
slideCell: "#banner",
titCell: ".hd ul", //开启自动分页 autoPage:true ,此时设置 titCell 为导航元素包裹层
mainCell: ".bd ul",
effect: "left",
autoPlay: true, //自动播放
autoPage: true, //自动分页
switchLoad: "_src" //切换加载,真实图片路径为"_src"
});
</script>
方法四:
借助swipe插件
注释:和touchsilde插件的效果一样
1.css代码:
<style type="text/css">
.swipe {
overflow: hidden;
visibility: hidden;
position: relative;
}
.swipe-wrap {
overflow: hidden;
position: relative;
}
.swipe-wrap > div {
float: left;
width: 100%;
position: relative;
}
.swipe-wrap > div img {
width: 100%;
height: 220px;
}
</style>
2.HTML代码:
<div id="slider" class="swipe">
<div class="swipe-wrap">
<div class="wrap">
<img src="img/3-1.jpg" />
</div>
<div class="wrap">
<img src="img/3-2.jpg" />
</div>
<div class="wrap">
<img src="img/3-3.jpg" />
</div>
</div>
</div>
3.js代码:
<script src="js/zepto.js"></script>
<script src="js/swipe.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.mySwipe = Swipe(document.getElementById("slider"), {
startSlide: 2,
speed: 400,
auto: 3000,
continuous: true,
disableScroll: false,
stopPropagation: false,
callback: function (index, elem) {},
transitionEnd: function (index, elem) {}
});
</script>
方法五:借助swipe插件 注释:该方法可以自动循环播放
1.swipe.css代码:
/*轮播*/
.carousel {
height: 250px;
position: relative;
}
.carousel .swipe {
overflow: hidden;
visibility: hidden;
position: relative;
height: 100%;
}
.carousel .swipe .swipe-wrap {
overflow: hidden;
position: relative;
}
.carousel .swipe .swipe-wrap > figure {
float: left;
width: 100%;
position: relative;
}
#slider {
max-width: 650px;
/* 设置最大的宽度 */
margin: 0px auto;
/* 居中对齐 */
}
figure {
margin: 0;
/* 对齐,四周宽度都为0,在轮播的时候,以整张图片进行移动 */
}
.face {
height: 250px;
/*设置图片高度*/
}
/*底边小点的设置*/
.carousel nav #position {
text-align: center;
list-style: none;
margin: 0;
padding: 0;
position: absolute;
bottom: 5px;
}
.carousel nav #position li {
display: inline-block;
width: 10px;
height: 10px;
border-radius: 10px;
background: #141414;
box-shadow: inset 0 1px 3px black, 0 0 1px 1px #202020;
margin: 0 2px;
cursor: pointer
}
.carousel nav #position li.on {
box-shadow: inset 0 1px 3px -1px #28b4ea, 0 1px 2px rgba(0, 0, 0, .5);
background-color: #1293dc;
background-image: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #1293dc), color-stop(100%, #0f6297));
background-image: -webkit-linear-gradient(top, #1293dc, #0f6297);
background-image: -moz-linear-gradient(top, #1293dc, #0f6297);
background-image: -ms-linear-gradient(top, #1293dc, #0f6297);
background-image: -o-linear-gradient(top, #1293dc, #0f6297);
background-image: linear-gradient(top, #1293dc, #0f6297)
}
2.html代码:
<div class="carousel">
<div id="slider" class="swipe" style="visibility: visible">
<div class="swipe-wrap">
<figure>
<div class="face faceInner">
<img src="img/4-1.jpg" width="100%" height="100%" />
</div>
</figure>
<figure>
<div class="face faceInner">
<img src="img/4-2.jpg" width="100%" height="100%" />
</div>
</figure>
<figure>
<div class="face faceInner">
<img src="img/4-3.jpg" width="100%" height="100%" />
</div>
</figure>
<figure>
<div class="face faceInner">
<img src="img/4-4.jpg" width="100%" height="100%" />
</div>
</figure>
</div>
</div>
<nav>
<ul id="position">
<li class="on"></li>
<li></li>
<li></li>
<li></li>
</ul>
</nav>
</div>
3.js代码:
<script src="js/swipe.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
var slider = Swipe(document.getElementById("slider"), {
auto: 3000, //轮播时间
continuous: true, //是否连续播放
disableScroll: false,
callback: function (pos) {
var i = bullets.length;
while (i--) {
bullets[i].className = " ";
}
bullets[pos].className = "on";
}
});
var bullets = document.getElementById("position").getElementsByTagName("li");
</script>