轮播图案例
2023-07-18 06:24
1.简单的轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>简单的轮播图</title>
<style>
ul {
list-style: none;
position: absolute;
margin: 0;
padding: 0;
width: 1000%;
}
img {
width: 400px;
height: 300px;
}
.box {
width: 400px;
height: 300px;
margin: 100px auto;
padding: 5px;
border: 1px solid gray;
}
.inner {
width: 400px;
height: 300px;
background-color: pink;
overflow: hidden;
position: relative;
}
.inner li {
float: left;
}
.square {
position: absolute;
right: 10px;
bottom: 10px;
}
.square span {
display: inline-block;
width: 16px;
height: 16px;
background-color: white;
text-align: center;
line-height: 16px;
cursor: pointer;
}
.square span.current {
background-color: orangered;
color: white;
}
</style>
</head>
<body>
<div class="box" id="box">
<div class="inner">
<!--相框-->
<ul>
<li>
<a href="#"><img src="images/1.jpg" /></a>
</li>
<li>
<a href="#"><img src="images/2.jpg" /></a>
</li>
<li>
<a href="#"><img src="images/3.jpg" /></a>
</li>
<li>
<a href="#"><img src="images/4.jpg" /></a>
</li>
<li>
<a href="#"><img src="images/5.jpg" /></a>
</li>
</ul>
<div class="square">
<span class="current">1</span>
<span>2</span>
<span>3</span>
<span>4</span>
<span>5</span>
</div>
</div>
</div>
<script>
function my$(id) {
return document.getElementById(id);
}
function animate(element, target) {
clearInterval(element.timeId);
element.timeId = setInterval(function () {
var current = element.offsetLeft;
var step = 9;
step = current < target ? step : -step;
current += step;
if (Math.abs(target - current) > Math.abs(step)) {
element.style.left = current + "px";
} else {
element.style.left = target + "px";
clearInterval(element.timeId);
}
}, 20);
}
//获取最外面的div
var box = my$("box");
//获取相框
var inner = box.children[0];
//获取相框的宽度
var imgWidth = inner.offsetWidth;
//获取ul
var ulObj = inner.children[0];
//获取所有span
var spanObjs = inner.children[1].children;
//循环遍历span,注册onmouseover
for (var i = 0; i < spanObjs.length; i++) {
spanObjs[i].setAttribute("index", i);
spanObjs[i].onmouseover = function () {
//排他,先去掉所有span的样式,为当前的span设置样式
for (var j = 0; j < spanObjs.length; j++) {
spanObjs[j].removeAttribute("class");
}
this.className = "current";
//移动ul(每个图片的宽*鼠标进入的span的索引)
var index = this.getAttribute("index");
animate(ulObj, -index * imgWidth);
};
}
</script>
</body>
</html>
鼠标进入对应的数字,显示相应的图片(图片移动)
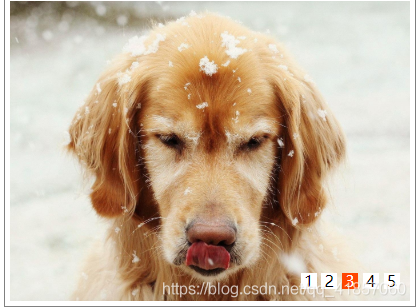
2.左右焦点轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>左右焦点轮播图</title>
<style>
ul {
list-style: none;
position: absolute;
margin: 0;
padding: 0;
width: 1000%;
}
img {
width: 400px;
height: 300px;
}
.box {
width: 400px;
height: 300px;
margin: 100px auto;
padding: 5px;
border: 1px solid gray;
position: relative;
}
.inner {
width: 400px;
height: 300px;
background-color: pink;
overflow: hidden;
position: relative;
}
.inner li {
float: left;
}
#focus {
display: none;
}
#focus span {
width: 40px;
height: 40px;
margin-top: -20px;
position: absolute;
left: 5px;
top: 50%;
background-color: black;
opacity: 0.3;
cursor: pointer;
line-height: 40px;
text-align: center;
font-weight: bold;
font-size: 30px;
color: white;
border: 1px solid white;
}
#focus #right {
right: 5px;
left: auto;
}
</style>
</head>
<body>
<div class="box" id="box">
<div class="inner">
<!--相框-->
<ul>
<li><img src="images/1.jpg" /></li>
<li><img src="images/2.jpg" /></li>
<li><img src="images/3.jpg" /></li>
<li><img src="images/4.jpg" /></li>
<li><img src="images/5.jpg" /></li>
</ul>
</div>
<div id="focus">
<span id="left"><</span><span id="right">></span>
</div>
</div>
<script>
function my$(id) {
return document.getElementById(id);
}
function animate(element, target) {
clearInterval(element.timeId);
element.timeId = setInterval(function () {
var current = element.offsetLeft;
var step = 9;
step = current < target ? step : -step;
current += step;
if (Math.abs(target - current) > Math.abs(step)) {
element.style.left = current + "px";
} else {
element.style.left = target + "px";
clearInterval(element.timeId);
}
}, 20);
}
var box = my$("box");
var inner = box.children[0];
var imgWidth = inner.offsetWidth;
var ulObj = inner.children[0];
//获取左右焦点的div
var focus = my$("focus");
//显示和隐藏左右焦点的div,为box注册事件
box.onmouseover = function () {
focus.style.display = "block";
};
box.onmouseout = function () {
focus.style.display = "none";
};
//点击右边按钮
var index = 0;
my$("right").onclick = function () {
if (index < ulObj.children.length - 1) {
index++;
animate(ulObj, -index * imgWidth);
}
};
//点击左边按钮
my$("left").onclick = function () {
if (index > 0) {
index--;
animate(ulObj, -index * imgWidth);
}
};
</script>
</body>
</html>
鼠标进入box时才显示左右两个按钮,点击按钮移动图片
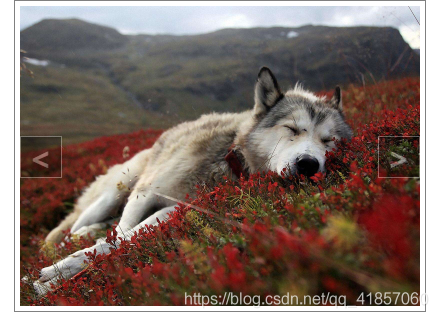
3.无缝轮播图
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8" />
<title>无缝轮播图</title>
<style>
ul {
list-style: none;
position: absolute;
margin: 0;
padding: 0;
width: 1000%;
left: 0;
top: 0;
}
img {
width: 400px;
height: 300px;
background-color: pink;
overflow: hidden;
position: relative;
}
.box {
width: 400px;
height: 300px;
margin: 100px auto;
background-color: pink;
border: 1px solid red;
position: relative;
overflow: hidden;
}
.box ul li {
float: left;
}
</style>
</head>
<body>
<div class="box" id="box">
<ul>
<li><img src="images/1.jpg" /></li>
<li><img src="images/2.jpg" /></li>
<li><img src="images/3.jpg" /></li>
<li><img src="images/4.jpg" /></li>
<li><img src="images/1.jpg" /></li>
<!--图片的第一张和最后一张是一样的,为了营造无缝效果-->
</ul>
</div>
<script>
function my$(id) {
return document.getElementById(id);
}
var current = 0; //只声明了一次
function f1() {
var ulObj = my$("box").children[0];
var imgWidth = Number(my$("box").offsetWidth) - 2;
//console.log(imgWidth);
current -= 10;
if (current < -imgWidth * (ulObj.children.length - 1)) {
//current<-400*4
//当前位置移动到最后一张图片时,立刻移动到第一张,无缝
ulObj.style.left = 0 + "px";
current = 0;
} else {
ulObj.style.left = current + "px";
}
}
var timeId = setInterval(f1, 20);
my$("box").onmouseover = function () {
//停止
clearInterval(timeId);
};
my$("box").onmouseout = function () {
//继续
timeId = setInterval(f1, 20);
};
</script>
</body>
</html>
鼠标进入,图片停止;移开,图片重新移动(实现无缝的要点:最后一张和第一张图片完全相同)
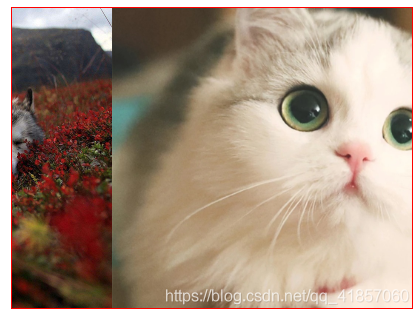
4.完整的轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>完整的轮播图</title>
<style>
ul {
list-style: none;
position: absolute;
margin: 0;
padding: 0;
width: 1000%;
}
img {
width: 400px;
height: 300px;
}
.box {
width: 400px;
height: 300px;
margin: 100px auto;
padding: 5px;
border: 1px solid gray;
position: relative;
}
.inner {
width: 400px;
height: 300px;
background-color: pink;
overflow: hidden;
position: relative;
}
.inner ol {
list-style: none;
position: absolute;
right: 10px;
bottom: 10px;
line-height: 20px;
text-align: center;
}
.inner li {
float: left;
}
.inner ol li {
width: 16px;
height: 16px;
line-height: 16px;
background-color: white;
border: 1px solid #ccc;
margin-left: 10px;
cursor: pointer;
}
.inner ol li.current {
background: orange;
color: white;
}
#focus {
display: none;
}
#focus span {
width: 40px;
height: 40px;
line-height: 40px;
margin-top: -20px;
position: absolute;
left: 5px;
top: 50%;
background-color: #000;
opacity: 0.3;
cursor: pointer;
text-align: center;
font-weight: bold;
font-size: 30px;
color: #fff;
border: 1px solid #fff;
}
#focus #right {
right: 5px;
left: auto;
}
</style>
</head>
<body>
<div class="box" id="box">
<div class="inner">
<!--相框-->
<ul>
<li><img src="images/1.jpg" /></li>
<li><img src="images/2.jpg" /></li>
<li><img src="images/3.jpg" /></li>
<li><img src="images/4.jpg" /></li>
<li><img src="images/5.jpg" /></li>
</ul>
<ol></ol>
<!--小按钮-->
</div>
<!--左右按钮-->
<div id="focus">
<span id="left"><</span><span id="right">></span>
</div>
</div>
<script>
function my$(id) {
return document.getElementById(id);
}
function animate(element, target) {
clearInterval(element.timeId);
element.timeId = setInterval(function () {
var current = element.offsetLeft;
var step = 9;
step = current < target ? step : -step;
current += step;
if (Math.abs(target - current) > Math.abs(step)) {
element.style.left = current + "px";
} else {
element.style.left = target + "px";
clearInterval(element.timeId);
}
}, 10);
}
//获取最外层div
var box = my$("box");
//获取相框
var inner = box.children[0];
//获取相框宽度
var imgWidth = inner.offsetWidth;
//获取ul
var ulObj = inner.children[0];
//获取ul中的所有的li
var list = ulObj.children;
//获取ol
var olObj = inner.children[1];
//左右按钮的div
var focus = my$("focus");
var pic = 0; //全局变量--鼠标进入的小按钮的索引
//创建小按钮----根据ul中的li个数
for (var i = 0; i < list.length; i++) {
//创建li标签,加入到ol中
var liObj = document.createElement("li");
olObj.appendChild(liObj);
liObj.innerHTML = i + 1;
//在每个ol中的li标签上添加一个自定义属性,存储索引值
liObj.setAttribute("index", i);
//设置ol中第一个li有背景颜色
olObj.children[0].className = "current";
//注册鼠标进入事件
liObj.onmouseover = function () {
//取消所有ol-li的类样式
for (var j = 0; j < olObj.children.length; j++) {
olObj.children[j].removeAttribute("class");
}
//设置当前鼠标进来的li的背景颜色
this.className = "current";
//获取鼠标进入的li的当前索引值
pic = this.getAttribute("index");
//移动ul
animate(ulObj, -pic * imgWidth);
};
}
//克隆ul中的第一个li,加入到ul中的最后---克隆(为了无缝)
ulObj.appendChild(list[0].cloneNode(true));
//自动播放--直接看成一直点击右按钮
var timeId = setInterval(clickHandle, 1000);
//鼠标进入,显示左右按钮的div
box.onmouseover = function () {
focus.style.display = "block";
//鼠标进入清除之前的定时器
clearInterval(timeId);
};
//鼠标离开,隐藏左右按钮的div
box.onmouseout = function () {
focus.style.display = "none";
//鼠标离开自动播放
timeId = setInterval(clickHandle, 1000);
};
//右边按钮
my$("right").onclick = clickHandle;
function clickHandle() {
//如果pic=5,此时页面显示第六个图片,而用户会认为这是第一个图,
//所以,如果用户再次点击按钮,用户应该看到第二个图片
if (pic === list.length - 1) {
//如何从第6个图,跳转到第1个图
pic = 0; //先设置pic=0
ulObj.style.left = pic + "px"; //把ul的位置还原成开始的默认位置
}
pic++; //立刻设置pic加1,那么此时用户就会看到第二个图片了
animate(ulObj, -pic * imgWidth); //pic从0的值加1之后,pic的值是1,然后ul移动出去一个图片
//如果pic=5说明,此时显示第6个图(内容是第一张图片),第一个小按钮有颜色
if (pic === list.length - 1) {
//第5个按钮颜色去掉样式
olObj.children[olObj.children.length - 1].className = "";
//为第1个按钮颜色设置样式
olObj.children[0].className = "current";
} else {
//去掉所有的小按钮的背景颜色
for (var i = 0; i < olObj.children.length; i++) {
olObj.children[i].removeAttribute("class");
}
olObj.children[pic].className = "current";
}
}
//左边按钮
my$("left").onclick = function () {
if (pic === 0) {
pic = list.length - 1;
ulObj.style.left = -pic * imgWidth + "px";
}
pic--;
animate(ulObj, -pic * imgWidth);
//设置小按钮的颜色--去掉所有的小按钮的颜色
for (var i = 0; i < olObj.children.length; i++) {
olObj.children[i].removeAttribute("class");
}
//当前的pic索引对应的按钮设置颜色
olObj.children[pic].className = "current";
};
</script>
</body>
</html>
效果是上面三个小案例的结合